How to Use the ChatGPT API in Python
Technology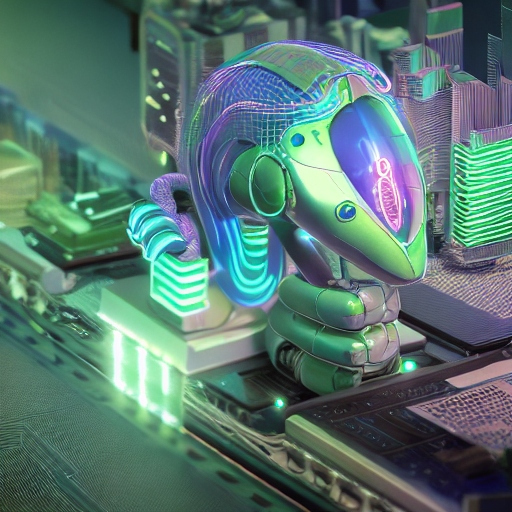
Image generated by deepai.org -- ChatGPT, Python, API, automatic, simple, AI, programmer
The first thing you need to do assuming you have python is to install openai. Using pip:
$ pip install openai
Now you can import openai into your python script. The os module is useful for reading getting OpenAI’s API key from a file or environment variable.
import openai
import os
from pprint import pp
# Need a key from openai.com but don't store the key in the script.
# Read the first line from the secret file where the key is kept.
with open('secret', 'r') as f:
txt = f.readline()
key = txt.strip()
# Define additional parameters for the API request
params = {
"engine": "text-davinci-003",
"temperature": 0.5,
"max_tokens": 500,
"top_p": 1,
"frequency_penalty": 0,
"presence_penalty": 0
}
# create a completion
def gpt3_response(prompt, key, params):
openai.api_key = key
# Print list of available models
models = openai.Model.list()
for model in models.data:
print(model.id)
completions = openai.Completion.create(
prompt=prompt,
engine=params["engine"],
temperature=params["temperature"],
max_tokens=params["max_tokens"],
top_p=params["top_p"],
frequency_penalty=params["frequency_penalty"],
presence_penalty=params["presence_penalty"],
)
return completions.choices[0].text
# print the completion
prompt = "Write a blog post on advantages there are to having ai write blog content."
response = gpt3_response(prompt, key, params)
pp(response)
As you can see it really is not very difficult. The hardest part was figuring out where to put the key. I have the key in my .bashrc but Emacs wasn’t reading the environment variables from Linux. Keeping the key in the script is not a good idea either.
My solution is to put the key in a file in the same directory as the script. If I start using git on the script I will just put the file in the .gitignore. Though I may need another solution if I start accessing the API more regularly in my code.
The output from running the script:
>>>
babbage
davinci
text-davinci-edit-001
babbage-code-search-code
text-similarity-babbage-001
code-davinci-edit-001
text-davinci-001
ada
curie-instruct-beta
babbage-code-search-text
babbage-similarity
whisper-1
code-search-babbage-text-001
text-curie-001
code-search-babbage-code-001
text-ada-001
text-embedding-ada-002
text-similarity-ada-001
ada-code-search-code
ada-similarity
text-davinci-003
code-search-ada-text-001
text-search-ada-query-001
davinci-search-document
ada-code-search-text
text-search-ada-doc-001
davinci-instruct-beta
text-similarity-curie-001
code-search-ada-code-001
ada-search-query
text-search-davinci-query-001
curie-search-query
gpt-3.5-turbo-0301
davinci-search-query
babbage-search-document
ada-search-document
text-search-curie-query-001
text-search-babbage-doc-001
gpt-3.5-turbo
curie-search-document
text-search-curie-doc-001
babbage-search-query
text-babbage-001
text-search-davinci-doc-001
text-search-babbage-query-001
curie-similarity
curie
text-similarity-davinci-001
text-davinci-002
davinci-similarity
cushman:2020-05-03
ada:2020-05-03
babbage:2020-05-03
curie:2020-05-03
davinci:2020-05-03
if-davinci-v2
if-curie-v2
if-davinci:3.0.0
davinci-if:3.0.0
davinci-instruct-beta:2.0.0
text-ada:001
text-davinci:001
text-curie:001
text-babbage:001
('\n'
'\n'
'If you’re a business owner or content creator looking to increase your '
'online presence, you’ve likely considered using artificial intelligence (AI) '
'to write blog content. AI-generated content can be a great way to keep your '
'blog fresh, save time, and reach a wider audience. Here are some of the '
'advantages of using AI to write blog content.\n'
'\n'
'1. Save Time: AI-generated content can be generated quickly and efficiently, '
'allowing you to focus your time and energy on other tasks. AI can also help '
'you to create content that is more targeted and relevant to your audience, '
'allowing you to get the most out of your blog posts.\n'
'\n'
'2. Reach a Wider Audience: AI-generated content can be tailored to the needs '
'and interests of your target audience, helping you to reach a wider '
'audience. AI can also help you to identify and target new markets, allowing '
'you to increase your reach and engagement.\n'
'\n'
'3. Create Unique Content: AI-generated content can help you to create unique '
'and interesting content that stands out from the competition. AI can also '
'help you to create content that is more engaging and informative, helping to '
'keep your readers interested and engaged.\n'
'\n'
'4. Automate Processes: AI-generated content can help you to automate '
'processes, allowing you to focus your time and energy on other tasks. AI can '
'also help you to identify trends and patterns in your content, allowing you '
'to adjust your content strategy accordingly.\n'
'\n'
'Overall, using AI to write blog content can be a great way to save time, '
'reach a wider audience, create unique content, and automate processes. If '
'you’re looking to increase your online presence and reach a larger audience, '
'AI-generated content can be a great way to do so.')
>>>